Lesson #4. Condition operator & branches. IF statement Part I (19/09)
if condition then some operator ...
some operator will be done if the condition is True
. If the condition is False
pascal will skip some operator.
var a:=5;
if a > 7 then
print(a-1)
print(a)
// the value of a (5) will be displayed
The statements after the if statement will run regardless if the condition is true or false:
print(a)
Full form of IF-statement:The else
statement runs a block of code if none of the conditions are True
before this else
statement:
if condition then operator1 else operator2
operator1 will be done if the condition is True
; in this case operator2 will be not executed. If the condition is False
pascal will skip operator1 and will execute operator2. Before else-block semicolon is not needed.
var a:=5; if a > 7 then print(a-1) else print(a+1) print(a) // at first the value of a+1 (6) will be displayed and then the value of a (5)
The semicolon before else
is not needed!
Also there can be not one but two or more conditions. Each condition must be in round brackets.
E.g:if(year<20) or (year>18) then … // if one of the conditions or both conditions are true then ...After if-block and else-block may go not one but some operators. In this case we have to use
begin..end
block:
if condition then begin operator1 operator2 end else begin operator3 operator4 end
The most popular errors:
else
.begin..end
in the cases when there is more than one operand after then
or else
.Examples
- If the variable is odd or even:
- If the variable is not greater than 2:
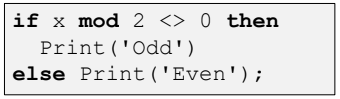

How to Swap Values of Variables?
1 solution:
2 solution:
Tasks (if-statement)
Max points for all tasks = 4
1. {0.5 points}[task-01-if.pas]
An integer is given. If it is positive then add 1 to it. Output the result.
-3 >>> -3 0 >>> 0 1 >>> 2 5 >>> 6
2. {0.5 points}[task-02-if.pas]
An integer is given. If it is even number then myltiply it by 10. Output the result.
2 >>> 20 1 >>> 1 -10 >>> -100
3. {0.5 points}[task-03-if.pas]
An integer is given. If it is even number then myltiply it by 3, if it is not even then devide it by 3. Output the result.
4 >>> 12 9 >>> 3 -10 >>> -30
4. {0.8 points}[task-04-if.pas]
An integer is given. If it is positive then add 1 to it; otherwise subtract it by 2. Output the number at the end of the program.
-3 >>> -5 0 >>> -2 1 >>> 2 5 >>> 6
5. {0.8 points}[task-05-if.pas]
An integer is given (the age of a person). If it is grater than or equal to 18 then output "you can watch this movie"; if the number is less than 10 then output "you should watch the cartoon". Otherwise output "you can take a walk".
18 >>> you can watch this movie 8 >>> you should watch the cartoon 15 >>> you can take a walk6. {0.8 points}
[task-06-if.pas]
Student has got a mark. If it is 2 mark then the program has to output "it's very bad"; if it is 3 - program has to output "it's bad"; if it is 4 - "it's good", in the case of 5 - "it's excellent", otherwise - "such marks do not exist" .
Example:2 >>> "it's very bad" 4 >>> "it's good"
7. {0.8 points}[task-07-if.pas]
The program must request the time of day in hours (from 1 up to 24). Depending on the time entered, display a message indicating what time of day the entered hour belongs to (midnight (24), night (1-4), morning (5-11), day (12-16), evening (17-13)).
Example:
2 >>> "night" 24 >>> "midnight"
8. {0.8 points}[task-08-if.pas]
Two integer values are given: for variables A
and B
. Assign a sum of these values to each variable if their values are not equal; otherwise (if equal) assign zero to the variables. Output new values of variables A
and B
.
A = -6, B = 10 >>> A = 4, B = 4 A = 2, B = 2 >>> A = 0, B = 0 A = 0, B = 0 >>> A = 0, B = 0
Check. Check the correctness of your program with at least two input sets: for the same and different values of the variables A
, B
. Give the log of the program with these sets in the form of a comment.
9. {0.3 points}[task-09-if.pas]
Two integer values are given: for variables A
and B
. Swap the values of the variables and output them.
A = -6, B = 10 >>> A = 10, B = -6
10. {0.5 points}[task-10-if.pas]
Two integer values are given: for variables A
and B
. If the value of A is greater than the value of B - swap the values of the variables and output them.
A = 6, B = 2 >>> A = 2, B = 6